The Input Panel serves as a containter for several input components such as textbox, combobox, checkbox, lable, etc. In order to get the value from a text box object, it must be searched from the Input Panel where it is attached.
The syntax for parsing input objects is: {InputPanelName.InputComponentName.FunctionName.FilterExpression.FormatEnum}
The syntax expression is separated by dot "." and each part is explained below:
InputPanelName = refers to the name of the input panel containter. For uniformity, always use InputPanel1. InputComponentName = refers to the name of the input component inside the input panel container FunctionName = refers to the function to be used when evaluating the value. The default function is "Value" which returns the value of the input object. FilterExpression = refers to the filter to be applied before retrieving the value of the control. Optional only. FormatEnum = refers to output format such as number format and date format. Optional only.
We will use the report app named FS - Basic as an example. The input objects will be used as paramaters in generating the trial balance.
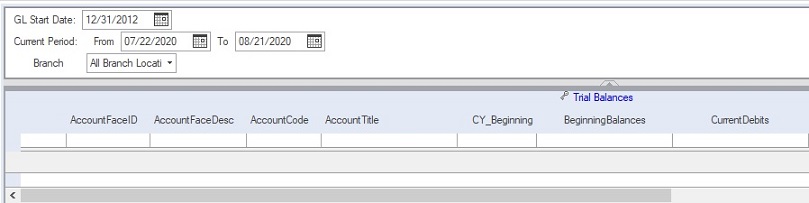
The following is the structure of the above input panel container.
Control Name
|
Display Value
|
Description
|
InputPanel1
|
|
The name of the control panel container. For uniformity, always use InputPanel1 as its name though some previously created input panels used c1InputPanel1.
|
DateStart
|
12/31/2012
|
The name of the input component with label GL Date Start
|
DateFr
|
01/01/2020
|
The name of the input component with label Current Period From
|
DateFr
|
06/30/2020
|
The name of the input component with label Current Period To
|
LocationID
|
All Branch Locations
|
The name of the input component with label Branch
|
The input panels come from the Input Panel Collections app. This is where you can see the full structure of an input panel.
|
Following are formula expressions to retrieve the values from the above input panel.
Formula Expression
|
Return Value
|
Description
|
{InputPanel1.DateStart} or {InputPanel1.DateStart.Value}
|
12/31/2012
|
InputPanel1 = the name of the input panel container. DateStart = the name of the Input Date Picker Value = the function name. This the default function so you can omit it.
|
{InputPanel1.DateFr}
|
01/01/2020
|
Returns the value of the object DateFr
|
{InputPanel1.DateTo.Value}
|
06/30/2020
|
Returns the value of the object DateTo
|
{c1InputPanel1.LocationID}
|
ALL
|
Returns the value of the object LocationID The actual value is "ALL" and not the display value of "All Branch Location" since this control contains dropdown value items.
|
Now, we can construct our Select Statement to generate the Trial Balances for the current transactions for the period 01/01/2020 - 06/30/2020 as follows: Focus only on the WHERE clause as this is only a code snippet.
SELECT * FROM vwgl_bookje WHERE CoID = '@CoID' AND (DocLocationID = '{c1InputPanel1.LocationID}' OR '{c1InputPanel1.LocationID}' = 'ALL') AND GLDate >= '{c1InputPanel1.DateFr}' AND GLDate <= '{c1InputPanel1.DateTo.Value}'
The parser will convert the above Select Statement as follows:
SELECT * FROM vwgl_bookje WHERE CoID = 'T10' AND (DocLocationID = 'ALL' OR 'ALL' = 'ALL') AND GLDate >= '01/01/2020' AND GLDate <= '06/30/2020'
The @CoID is one of the global variables that represents the current Company ID that the user is currently log-in. See Global Variables for details.
Most of the time, you will need only the value of the input object as discussed above. For further references, below is the actual code used by the system.
/// /// Returns evaluated value, usually the text value of an InputComonent. /// If SqlValues are to be returned, the ff format will be used "('value1','value2','etc') /// Morover, the InputComponent's name shall be used for retrieving values from lookupViewer in case multiple selection's is made /// public static T ecGetEvaluatedValue(this C1InputPanel inputPanelObject, string inputComponentName, string funcName, string filterExp, string formatEnum) { string retVal = string.Empty; //find inputComponent foreach (InputComponent ic in inputPanelObject.Items) { if (ic.Name.ToLower().Trim() == inputComponentName.ToLower().Trim()) { if (funcName.Trim().ToLower() == "length") retVal = ic.Text.Trim().Length.ToString(); else if (funcName.Trim().ToLower() == "encryptedvalue") retVal = ecFunction.PasswordEncryption(ic.Text.Trim()); else if (funcName.ecIsSqlWhereInPropertyType() || funcName.ecIsParserPropertyType()) { DataTable dt = ic.ecGetHashTableValueFromTagProperty("MultipleSelection"); if (dt != null && dt.Rows.Count > 0) { //multiple rows were selected; used ic.Name as the columnName and filtExp as the defaultType retVal = dt.DefaultView.ecGetColumnValuesToStringArray(ic.Name, "").ecToStringSqlWhereInClauseFormat(dt.ecGetColumnDataType(ic.Name), filterExp); } else //single selection is made only retVal = ic.Text.ecToSqlWhereInClauseFormat(ic.GetType().UnderlyingSystemType.Name, filterExp, null);
//format further for SqlValues by adding "\" before each single quote if (funcName.ecIsParserPropertyType()) { retVal = retVal.ecConvertSqlWhereInToParserFormat(); } }
else if (ic is InputComboBox) { InputComboBox cb = (InputComboBox)ic; if (cb.SelectedValue == null) retVal = cb.Text; else retVal = cb.SelectedValue.ToString(); } else if (ic is InputCheckBox) { InputCheckBox cb = (InputCheckBox)ic; if (cb.Checked) retVal = "True"; else retVal = "False"; } else if (ic is InputRadioButton) { InputRadioButton cb = (InputRadioButton)ic; if (cb.Checked) retVal = "True"; else retVal = "False"; } else if (ic is InputDatePicker) { InputDatePicker cb = (InputDatePicker)ic; if (formatEnum==null || formatEnum.Length == 0) retVal = cb.Value.ToString("MM/dd/yyyy"); else retVal = cb.Value.ToString(formatEnum); //retVal = ic.Text; //default proc name is Text } else retVal = ic.Text; //default proc name is Text // //set default value for empty string if (retVal.Length == 0) if (ic is InputNumericBox) retVal = "0"; //default value for empty string //already found, exit foreach now break; } } ////evaluate further TO BE DONE BY ecParser instead //if (formatEnum != null && formatEnum.Length > 0) // retVal = retVal.ecToParserEvaluatedFormatEnum(formatEnum); //// return (T)Convert.ChangeType(retVal, typeof(T));
}
|