The Pagination Parameter is a pop-up window shows before the main app. It's usually used to ask for query parameters to be passed to the calling app.
The syntax for parsing pagination parameter is: {Pagination.InputComponentName.FunctionName.FilterExpression.FormatEnum}
The syntax expression is separated by dot "." and each part is explained below:
Pagination = refers to the name of the input panel containter of the pagination query viewer. InputComponentName = refers to the name of the input object inside the input panel container FunctionName = refers to the function to be used when evaluating the value. The default function is "Value" which returns the value of the input object. Optional only. FilterExpression = refers to the filter to be applied before retrieving the value of the control. Optional only. FormatEnum = refers to output format such as number format and date format. Optional only.
Below is a sample of pagination used for Systems -> GL -> Journal Entry v20. It requires the user to input the Covering Period before the Journal Entry app is executed.
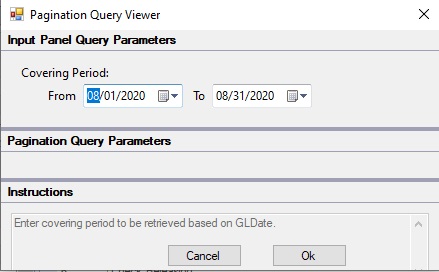
The following is the structure of the above Pagination Parameter
Control Name
|
Display Value
|
Description
|
Pagination
|
|
The name of the input panel container inside the pagination query viewer.
|
DateFr
|
08/01/2020
|
The name of the input component with label "From"
|
DateFr
|
08/31/2020
|
The name of the input component with label "To"
|
Since you will be using the App Gallery when creating your formula, you can easily see the names of these input objects. As much as possible, use the same cotrol name for your input objects so you can use them interchangeably.
|
Following are formula expressions to retrieve the values from the above pagination query viewer
Formula Expression
|
Return Value
|
Description
|
{Pagination.DateFr}
|
01/01/2020
|
Returns the value of the object DateFr
|
{Pagination.DateTo}
|
06/30/2020
|
Returns the value of the object DateTo
|
The FunctionName, FilterExpression and FormatEnum are optional only. Most of the time you only need the value of the input component.
|
Now, we can construct our Select Statement to retrieve journal entries for the period the period 08/01/2020 - 08/31/2020 as follows: Focus only on the WHERE clause as this is only a code snippet.
SELECT * FROM gl_BookJE WHERE CoID = '@CoID' AND GLDate >= '{c1InputPanel1.DateFr}' AND GLDate <= '{c1InputPanel1.DateTo.Value}'
The parser will convert the above Select Statement as follows:
SELECT * FROM vwgl_bookje WHERE CoID = 'T10' AND GLDate >= '08/01/2020' AND GLDate <= '08/31/2020'
The @CoID is one of the global variables that represents the current Company ID that the user is currently log-in. See Global Variables for details.
The Input Panel Query Parameters section uses input panels from Input Panel Collections app.
The same code is used for parsing both Pagination Parameter and Input Panels as shown below:
/// /// Returns evaluated value, usually the text value of an InputComonent. /// If SqlValues are to be returned, the ff format will be used "('value1','value2','etc') /// Morover, the InputComponent's name shall be used for retrieving values from lookupViewer in case multiple selection's is made /// public static T ecGetEvaluatedValue(this C1InputPanel inputPanelObject, string inputComponentName, string funcName, string filterExp, string formatEnum) { string retVal = string.Empty; //find inputComponent foreach (InputComponent ic in inputPanelObject.Items) { if (ic.Name.ToLower().Trim() == inputComponentName.ToLower().Trim()) { if (funcName.Trim().ToLower() == "length") retVal = ic.Text.Trim().Length.ToString(); else if (funcName.Trim().ToLower() == "encryptedvalue") retVal = ecFunction.PasswordEncryption(ic.Text.Trim()); else if (funcName.ecIsSqlWhereInPropertyType() || funcName.ecIsParserPropertyType()) { DataTable dt = ic.ecGetHashTableValueFromTagProperty("MultipleSelection"); if (dt != null && dt.Rows.Count > 0) { //multiple rows were selected; used ic.Name as the columnName and filtExp as the defaultType retVal = dt.DefaultView.ecGetColumnValuesToStringArray(ic.Name, "").ecToStringSqlWhereInClauseFormat(dt.ecGetColumnDataType(ic.Name), filterExp); } else //single selection is made only retVal = ic.Text.ecToSqlWhereInClauseFormat(ic.GetType().UnderlyingSystemType.Name, filterExp, null);
//format further for SqlValues by adding "\" before each single quote if (funcName.ecIsParserPropertyType()) { retVal = retVal.ecConvertSqlWhereInToParserFormat(); } }
else if (ic is InputComboBox) { InputComboBox cb = (InputComboBox)ic; if (cb.SelectedValue == null) retVal = cb.Text; else retVal = cb.SelectedValue.ToString(); } else if (ic is InputCheckBox) { InputCheckBox cb = (InputCheckBox)ic; if (cb.Checked) retVal = "True"; else retVal = "False"; } else if (ic is InputRadioButton) { InputRadioButton cb = (InputRadioButton)ic; if (cb.Checked) retVal = "True"; else retVal = "False"; } else if (ic is InputDatePicker) { InputDatePicker cb = (InputDatePicker)ic; if (formatEnum==null || formatEnum.Length == 0) retVal = cb.Value.ToString("MM/dd/yyyy"); else retVal = cb.Value.ToString(formatEnum); //retVal = ic.Text; //default proc name is Text } else retVal = ic.Text; //default proc name is Text // //set default value for empty string if (retVal.Length == 0) if (ic is InputNumericBox) retVal = "0"; //default value for empty string //already found, exit foreach now break; } } ////evaluate further TO BE DONE BY ecParser instead //if (formatEnum != null && formatEnum.Length > 0) // retVal = retVal.ecToParserEvaluatedFormatEnum(formatEnum); //// return (T)Convert.ChangeType(retVal, typeof(T));
}
|